C++ allows you to specify more than one definition for a function name or an operator in the same scope, which is called function overloading and operator overloading respectively.
It is a type of polymorphism in which an operator is overloaded to give user defined meaning to it. Overloaded operator is used to perform operation on user-defined data type.
Operator that are not overloaded are follows
- – scope operator –
::
- sizeof
- – member selector –
.
- – member pointer selector –
*
- – ternary operator –
?:
C++ Operator Overload Following are some restrictions to be kept in mind while implementing operator overloading.
- – Precedence and Associativity of an operator cannot be changed.
- – Arity (numbers of Operands) cannot be changed. Unary operator remains unary, binary remains binary etc.
- – No new operators can be created, only existing operators can be overloaded.
- – Cannot redefine the meaning of a procedure. You cannot change how integers are added.
For example,
We can overload an operator ‘+’ in a class like String so that we can concatenate two strings by just using +.
#include<iostream>
using namespace std;
class Complex
{
private:
int real, imag;
public:
Complex(int r = 0, int i =0) {real = r; imag = i;}
void print()
{
cout << real << ” + i” << imag << endl;
}
friend Complex operator + (Complex const &, Complex const &);
};
Complex operator + (Complex const &c1, Complex const &c2)
{
return Complex(c1.real + c2.real, c1.imag + c2.imag);
}
int main()
{
Complex c1(10, 5), c2(2, 4);
Complex c3 = c1 + c2;
c3.print();
return 0;
}
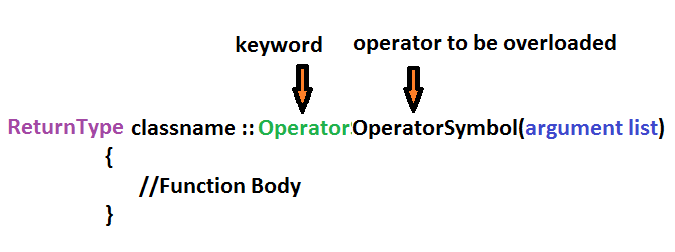